java.lang包下的Exception如下:
Exception
-ClassNotFoundException
-CloneNotSupportedException
-
IllegalAccessException
-InstantiationException
-InterruptedException
-NoSuchFieldException
-NoSuchMethodException
RuntimeException extends
Exception
-ArithmeticException
-IndexOutOfBoundsException
-ArrayIndexOutOfBoundsException
-StringIndexOutOfBoundsException
-ArrayStoreException
-ClassCastException
-
EnumConstantNotPresentException
-IllegalArgumentException
-IllegalThreadStateException
-NumberFormatException
-IllegalMonitorStateException
-
IllegalStateException
-IndexOutOfBoundsException
-NegativeArraySizeException
-
SecurityException
-
NullPointerException
-
TypeNotPresentException
Exception的描述如下:
以下给出
异常产生的实例,红色标记的暂时还没有提供
例子。
ArithmeticException
class="java">package my.exception;
public class ArithmeticExceptionTest {
public static void main(String[] args) {
int a = 10;
a = a/0;
}
}
ArrayIndexOutOfBoundsException
package my.exception;
public class ArrayIndexOutOfBoundsExceptionExample {
public static void main(String[] args) {
int[] array = {1,2,3};
System.out.println(array[3]);
}
}
ArrayStoreException
package my.exception;
import java.util.HashMap;
import java.util.Map;
public class ArrayStoreExceptionExample {
public static void main(String[] args) {
Map<String, Object> map = new HashMap<String, Object>();
map.put("数字", 123);
map.put("字符", "Java");
Object[] valueArr = map.values().toArray(new String[map.size()]);
for(int i = 0;i<valueArr.length;i++){
System.out.println(valueArr[i]);
}
}
}
ClassCastException
package my.exception;
public class ClassCastExceptionExample {
public static void main(String[] args) {
Object x = new Integer(0);
System.out.println((String)x);
}
}
ClassNotFoundException
package my.exception;
public class ClassNotFoundExceptionExample {
public static void main(String[] args) throws ClassNotFoundException {
Class c = Class.forName("my.exception.ClassNotFoundExceptionExample1");
}
}
CloneNotSupportedException
package my.exception;
public class CloneNotSupportedExceptionExample {
public static void main(String[] args) throws CloneNotSupportedException {
CloneNotSupportedExceptionExample c2 = (CloneNotSupportedExceptionExample) new CloneNotSupportedExceptionExample()
.clone();
}
}
IllegalArgumentException
package my.exception;
public class IllegalArgumentExceptionExample {
public static void main(String[] args) {
//Work fine
Season s = Enum.valueOf(Season.class, "SPRING");
//Throws IllegalArgumentException
Season s1 = Enum.valueOf(Season.class, "SPRING111");
}
}
enum Season
{
SPRING,SUMMER,AUTUMN,WINTER;
}
IllegalMonitorStateException
package my.exception;
public class IllegalMonitorStateExceptionExample {
public static void main(String[] args) throws InterruptedException {
Thread test = new Thread();
test.start();
test.wait();
test.countStackFrames();
}
}
IllegalThreadStateException
package my.exception;
public class IllegalThreadStateException {
public static void main(String[] args) {
Thread test = new Thread();
//Thread can not be started twice. If we do, then an IllegalThreadStateException occurs.
test.start();
test.start();
}
}
InstantiationException
package my.exception;
public class InstantiationExceptionExample {
static Object createNewInstance(Object obj) {
try {
return obj.getClass().newInstance();
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
return null;
}
public static void main(String[] args) {
String[] s = new String[] { "a", "b", "c" };
createNewInstance(s);
}
}
InterruptedException
package my.exception;
public class InterruptedExceptionExample {
public static void main(String[] args) throws InterruptedException {
final Thread t1 = new Thread() {
public void run() {
try {
Thread.sleep(10000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
};
Thread t2 = new Thread() {
public void run() {
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
t1.interrupt();
}
};
t1.start();
t2.start();
}
}
NegativeArraySizeException
package my.exception;
public class NegativeArraySizeExceptionExample {
public static void main(String[] args) {
int[] array = new int[-1];
}
}
NoSuchFieldException
package my.exception;
import java.lang.reflect.Field;
public class NoSuchFieldException {
public static void main(String[] args) throws Exception {
Field field = NoSuchFieldException.class.getField("name");
}
}
NoSuchMethodException
public class NoSuchMethodExceptionExample {
public static void main(String[] args) {
try {
NoSuchMethodExceptionExample.class.getMethod("getName", String.class);
} catch (SecurityException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (NoSuchMethodException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
NullPointerException
package my.exception;
public class NullPointerExceptionExample {
public static void main(String[] args) {
String name = null;
System.out.println(name.toLowerCase());
}
}
NumberFormatException
package my.exception;
public class NumberFormatExceptionExample {
public static void main(String[] args) {
int value = Integer.valueOf("123.25f");
}
}
StringIndexOutOfBoundsException
package my.exception;
public class StringIndexOutOfBoundsExceptionExample {
public static void main(String[] args) {
String value = "hello world!";
char c = value.charAt(20);
}
}
UnsupportedOperationException
package my.exception;
import java.util.Arrays;
import java.util.List;
public class UnsupportedOperationExceptionExample {
public static void main(String[] args) {
String[] array = {"Hello","World","Java"};
List<String> test = Arrays.asList(array);
//Arrays.asList获取的list是不能用于删除的
test.remove("Hello");
}
}
对于上述标红的,暂时没有例子的异常,博友们有直接产生的例子也请共享哦。
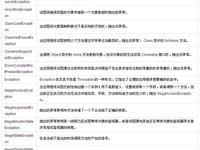
- 大小: 109.1 KB
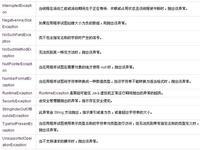
- 大小: 68.3 KB