本文
结合File,FileInputStream,FileRedaer以及BufferedReader的方法,将给出四个判断文件是否为空的方法:
本文包括如下三个部分:
1. 贴出File,FileInputStream,FileRedaer以及BufferedReader相应方法的源代码,了解一下。
2. 给出自己的实现。
3. 测试并给出分析结论。
File,FileInputStream,FileRedaer以及BufferedReader相应方法的源代码
class="java">/**
* Returns the length of the file denoted by this abstract pathname.
* The return value is unspecified if this pathname denotes a directory.
*
* @return The length, in bytes, of the file denoted by this abstract
* pathname, or <code>0L</code> if the file does not exist
*
* @throws SecurityException
* If a security manager exists and its <code>{@link
* java.lang.SecurityManager#checkRead(java.lang.String)}</code>
* method denies read access to the file
*/
public long length() {
SecurityManager security = System.getSecurityManager();
if (security != null) {
security.checkRead(path);
}
return fs.getLength(this);
}
public
class FileInputStream extends InputStream
{
/**
* Returns the number of bytes that can be read from this file input
* stream without blocking.
*
* @return the number of bytes that can be read from this file input
* stream without blocking.
* @exception IOException if an I/O error occurs.
*/
public native int available() throws IOException;
}
public class InputStreamReader extends Reader {
/**
* Tell whether this stream is ready to be read. An InputStreamReader is
* ready if its input buffer is not empty, or if bytes are available to be
* read from the underlying byte stream.
*
* @exception IOException If an I/O error occurs
*/
public boolean ready() throws IOException {
return sd.ready();
}
}
public class FileReader extends InputStreamReader {
}
public class BufferedReader extends Reader {
/**
* Tell whether this stream is ready to be read. A buffered character
* stream is ready if the buffer is not empty, or if the underlying
* character stream is ready.
*
* @exception IOException If an I/O error occurs
*/
public boolean ready() throws IOException {
synchronized (lock) {
ensureOpen();
/*
* If newline needs to be skipped and the next char to be read
* is a newline character, then just skip it right away.
*/
if (skipLF) {
/* Note that in.ready() will return true if and only if the next
* read on the stream will not block.
*/
if (nextChar >= nChars && in.ready()) {
fill();
}
if (nextChar < nChars) {
if (cb[nextChar] == '\n')
nextChar++;
skipLF = false;
}
}
return (nextChar < nChars) || in.ready();
}
}
}
给出自己的实现类
package my.tool.file.util;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
public class EmptyFileChecker {
public static boolean isFileEmpty1(File file) {
FileInputStream fis = null;
boolean flag = true;
try {
fis = new FileInputStream(file);
if (fis.available() != 0) {
flag = false;
}
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if (null != fis) {
try {
fis.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
fis = null;
}
}
}
return flag;
}
public static boolean isFileEmpty2(File file) {
boolean flag = true;
FileReader fr = null;
try {
fr = new FileReader(file);
if (fr.ready()) {
flag = false;
}
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if (null != fr) {
try {
fr.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
fr = null;
}
}
}
return flag;
}
public static boolean isFileEmpty3(File file) {
boolean flag = true;
BufferedReader br = null;
try {
br = new BufferedReader(new FileReader(file));
if (br.ready()) {
flag = false;
}
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if (null != br) {
try {
br.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
br = null;
}
}
}
return flag;
}
public static boolean isFileEmpty4(File file) {
return file.length() ==0L;
}
}
为了测试文件是否为空,新建多种文件类型的文件。
Note:
新建文件后,像zip文件它们的大小都不是0KB。
编写一个测试类:
package my.tool.file.util;
import java.io.File;
public class Main {
public static void main(String[] args) {
for(File file: new File("D: \\EmptyFileFolder").listFiles())
{
System.out.print(file.getName());
System.out.print(" ");
System.out.print(" is empty ? ");
System.out.println();
System.out.println("Call method available in FileInputStream -->" + EmptyFileChecker.isFileEmpty1(file));
System.out.println("Call method ready in FileReader -->" + EmptyFileChecker.isFileEmpty2(file));
System.out.println("Call method ready in BufferedReader -->" + EmptyFileChecker.isFileEmpty3(file));
System.out.println("Call method length in File -->" + EmptyFileChecker.isFileEmpty4(file));
System.out.println();
}
}
}
输出的结果如下:
新建 BMP 图像.bmp is empty ?
Call method available in FileInputStream -->true
Call method ready in FileReader -->true
Call method ready in BufferedReader -->true
Call method length in File -->true
新建 Microsoft
Office Access 2007 Database.accdb is empty ?
Call method available in FileInputStream -->false
Call method ready in FileReader -->false
Call method ready in BufferedReader -->false
Call method length in File -->false
新建 Microsoft Office Word Document.docx is empty ?
Call method available in FileInputStream -->true
Call method ready in FileReader -->true
Call method ready in BufferedReader -->true
Call method length in File -->true
新建 OpenDocument Drawing.odg is empty ?
Call method available in FileInputStream -->false
Call method ready in FileReader -->false
Call method ready in BufferedReader -->false
Call method length in File -->false
新建 OpenDocument
Spreadsheet.ods is empty ?
Call method available in FileInputStream -->false
Call method ready in FileReader -->false
Call method ready in BufferedReader -->false
Call method length in File -->false
新建 压缩(zipped)文件夹.zip is empty ?
Call method available in FileInputStream -->false
Call method ready in FileReader -->false
Call method ready in BufferedReader -->false
Call method length in File -->false
新建 文本文档.txt is empty ?
Call method available in FileInputStream -->true
Call method ready in FileReader -->true
Call method ready in BufferedReader -->true
Call method length in File -->true
新建 波形声音.wav is empty ?
Call method available in FileInputStream -->false
Call method ready in FileReader -->false
Call method ready in BufferedReader -->false
Call method length in File -->false
结论: 通过File的length方法,FileInputStream的available方法或者是FileReader以及BufferedReader的ready方法,只有当新建的文件是0KB的时候,才能正确判断文件是否为空。如果新建后的文件有一定的大小,比如zip文件有1KB,这样的文件,如果想要知道文件里面有没有内容,只能采用其它方式来做。
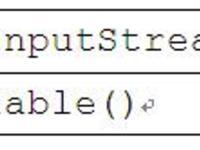
- 大小: 19.7 KB
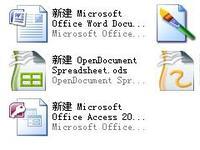
- 大小: 21.9 KB
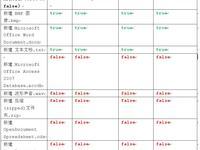
- 大小: 96.8 KB