[list]
控制器的Action方法注解写法更新.
原来写法:
@RequestMapping(value="helloWorld", method="GET|POST", showValErr="dwzJson")
public void hello(PrintWriter out){
out.print("hello world!");
}
现在写法:
@Path("helloWorld")
@GET
@POST
@ShowValMess("dwzJson")
public void hello(PrintWriter out){
out.print("hello world!");
}
新增验证器注解写法
@Controller
@Path("users")
public class UserControlTest {
@Required
@IDCard
private long idCard;// 身份证号码,必填
@Required
@Chinese
private String chineseName;// 中文姓名,必填
@Date(format="yyyy年MM月dd号")
private String birthDay;//出生日期,格式:yyyy年MM月dd号
@Int
@Size(min = 18, max = 50)
private int age = 0;// 年龄 0 为保密
@Required
@Length(min = 6, max = 18)
private String account;// 帐号,必填
@Required
@Length(min = 6, max = 32)
private String password;// 密码,必填
@Equals(to="password", mess="请确认密码")
private String confirmPwd;// 确认密码
@Email
private String email;// 邮件地址
@QQ
private String qq;// QQ号码
@Enum(words = { "男", "女", "保密" })
private String gender;// 性别
@Length(min = 50, max = 500)
@Forbid(words = { "独立", "反gong", "性爱" })
private String intro;// 个人简介,要求文明用语
@Path("profile")
@GET
@ShowValMess("input.jsp")
// 验证信息在显示
public void showProfile(PrintWriter out) {
out.print(this);
}
@Override
public String toString() {
return "User [idCard=" + idCard + ", chineseName=" + chineseName
+ ", birthDay=" + birthDay + ", age=" + age + ", account="
+ account + ", password=" + password + ", confirmPwd="
+ confirmPwd + ", email=" + email + ", qq=" + qq + ", gender="
+ gender + ", intro=" + intro + "]";
}
}
测试页面input.jsp:
<%@ page contentType="text/html; charset=utf-8" language="java" import="java.util.*" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>欢迎使用EWeb4J框架 !</title>
<style>
*{ font-family:Verdana, Geneva, sans-serif; margin:0; padding:0; font-size:12px; line-height:12px;}
.unit{ margin:0 auto;width:500px; padding:10px;}
.unit span{padding:5px; color:red; display:block; text-align:center;}
label{ display:inline-block; padding:10px; padding-right:20px; width:100px; text-align:right; font-size:14px;}
input,select{width:250px;height:30px;}
.button{width:50px;}
</style>
</head>
<body>
<form action="profile" method="get">
<div class="unit">
<label>身份证号码:</label>
<input type="text" name="idCard" value="${idCard[0]}" />
<span>${valError.idCard}</span>
</div>
<div class="unit">
<label>中文姓名:</label>
<input type="text" name="chineseName" value="${chineseName[0]}" />
<span>${valError.chineseName}</span>
</div>
<div class="unit">
<label>出生日期:</label>
<input type="text" name="birthDay" value="${birthDay[0]}" />
<span>${valError.birthDay}</span>
</div>
<div class="unit">
<label>年龄:</label>
<input type="text" name="age" value="${age[0]}" />
<span>${valError.age}</span>
</div>
<div class="unit">
<label>帐号:</label>
<input type="text" name="account" value="${account[0]}" />
<span>${valError.account}</span>
</div>
<div class="unit">
<label>密码:</label>
<input type="password" name="password" value="${password[0]}" />
<span>${valError.password}</span>
</div>
<div class="unit">
<label>确认密码:</label>
<input type="password" name="confirmPwd" value="${confirmPwd[0]}" />
<span>${valError.confirmPwd}</span>
</div>
<div class="unit">
<label>邮件地址:</label>
<input type="text" name="email" value="${email[0]}" />
<span>${valError.email}</span>
</div>
<div class="unit">
<label>QQ:</label>
<input type="text" name="qq" value="${qq[0]}" />
<span>${valError.qq}</span>
</div>
<div class="unit">
<label>性别:</label>
<select name="gender">
<option selected="selected">保密</option>
<option>男</option>
<option>女</option>
<option>人妖</option>
</select>
<span>${valError.gender}</span>
</div>
<div class="unit">
<label>个人简介:</label>
<textarea name="intro" rows="8" cols="50" >${intro[0]}</textarea>
<span>${valError.intro}</span>
</div>
<div class="unit" >
<center>
<input class="button" type="submit" value="提交" />
<input class="button" type="reset" value="重置" /></center>
</div>
</form>
</body>
</html>
eweb没有自己写
标签库,验证器验证得到的提示信息将会保存在request或session中,保存的key为"valError",它是一个map,所有的
错误信息以字段名字为key进行保存.因此在jsp上可以这样来写:request.getAttribute("valError"); 不过我们肯定使用EL
表达式来取数据啦.
eweb新的验证器默认有提示信息,当开发者不显示提供提示信息内容,就会采用,下面列出这些默认的信息内容.
@chinese // 请填写全中文
@Date(format="yyMMdd") // 请填写正确的日期格式:yyMMdd
@Email // 请填写正确的Email格式,例如:yourname@domain.com
@Enum(words={"a","b"}) // 请输入下列的值:[a#b]
@Equals(to="xxx") // 请确认{值}
@Forbid(words={"a","b"}) // 请不要包含下列字眼:[a#b]
@IDCard // 请填写正确的身份证号码,例如:441625198306051616
@Int // 请填写正确的整数,例如:5
@IP // 请填写正确的IP地址,例如:192.168.0.1
@Length(min=2,max=5) // 请填写一个长度介于 2 到 5 之间的字符串
@Phone // 请填写正确的家庭固话号码,例如0759-3365542
@QQ // 请填写正确的QQ号码,例如549189557
@Regex(pattren="xx") // 请填写匹配以下正则表达式的值:xx
@Required // 请完成必填内容
@Size(min=2,max=5) // 请填写一个大小介于 2 到 5 之间的整数
@Url // 请填写正确的URL,例如:http://www.google.com
@Zip // 请填写正确的邮编号码,例如:524088
部署到tomcat,打开input.jsp:
然后什么都不填写,提交:
接着随便输入,提交:
最后,全部填写正确,提交:
几天暂时就这么多,最近在实习中,抱歉没有更新.
最新更新了一个重复值的验证:
[/list]
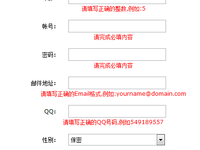
- 大小: 11.8 KB
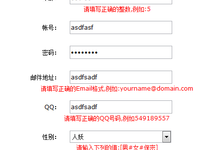
- 大小: 15.3 KB
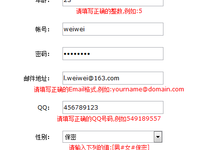
- 大小: 17.9 KB
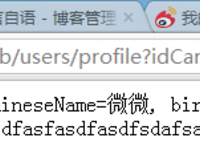
- 大小: 23.8 KB
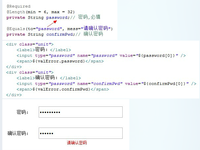
- 大小: 141 KB