class="Apple-converted-space"> C#中利用GDI+绘制旋转文本的文字,网上有很多资料,基本都使用矩阵旋转的方式实现。但基本都只提及按点旋转,若要实现在矩形范围内旋转文本,资料较少。经过琢磨,可以将矩形内旋转转化为按点旋转,不过需要经过不少的计算过程。利用下面的类可以实现该功能。
[csharp] view plaincopy
- using System;
- using System.Collections.Generic;
- using System.Drawing;
- using System.Drawing.Drawing2D;
-
- namespace RotateText
- {
- public class GraphicsText
- {
- private Graphics _graphics;
-
- public GraphicsText()
- {
-
- }
-
- public Graphics Graphics
- {
- get { return _graphics; }
- set { _graphics = value; }
- }
-
-
-
-
-
-
-
-
-
-
- public void DrawString(string s, Font font, Brush brush, RectangleF layoutRectangle, StringFormat format, float angle)
- {
-
- SizeF size = _graphics.MeasureString(s, font);
-
-
- SizeF sizeRotate = ConvertSize(size, angle);
-
-
- PointF rotatePt = GetRotatePoint(sizeRotate, layoutRectangle, format);
-
-
- StringFormat newFormat = new StringFormat(format);
- newFormat.Alignment = StringAlignment.Center;
- newFormat.LineAlignment = StringAlignment.Center;
-
-
- DrawString(s, font, brush, rotatePt, newFormat, angle);
- }
-
-
-
-
-
-
-
-
-
-
- public void DrawString(string s, Font font, Brush brush, PointF point, StringFormat format, float angle)
- {
-
- Matrix mtxSave = _graphics.Transform;
-
- Matrix mtxRotate = _graphics.Transform;
- mtxRotate.RotateAt(angle, point);
- _graphics.Transform = mtxRotate;
-
- _graphics.DrawString(s, font, brush, point, format);
-
-
- _graphics.Transform = mtxSave;
- }
-
- private SizeF ConvertSize(SizeF size, float angle)
- {
- Matrix matrix = new Matrix();
- matrix.Rotate(angle);
-
-
- PointF[] pts = new PointF[4];
- pts[0].X = -size.Width / 2f;
- pts[0].Y = -size.Height / 2f;
- pts[1].X = -size.Width / 2f;
- pts[1].Y = size.Height / 2f;
- pts[2].X = size.Width / 2f;
- pts[2].Y = size.Height / 2f;
- pts[3].X = size.Width / 2f;
- pts[3].Y = -size.Height / 2f;
- matrix.TransformPoints(pts);
-
-
- float left = float.MaxValue;
- float right = float.MinValue;
- float top = float.MaxValue;
- float bottom = float.MinValue;
-
- foreach(PointF pt in pts)
- {
-
- if(pt.X < left)
- left = pt.X;
- if(pt.X > right)
- right = pt.X;
- if(pt.Y < top)
- top = pt.Y;
- if(pt.Y > bottom)
- bottom = pt.Y;
- }
-
- SizeF result = new SizeF(right - left, bottom - top);
- return result;
- }
-
- private PointF GetRotatePoint(SizeF size, RectangleF layoutRectangle, StringFormat format)
- {
- PointF pt = new PointF();
-
- switch (format.Alignment)
- {
- case StringAlignment.Near:
- pt.X = layoutRectangle.Left + size.Width / 2f;
- break;
- case StringAlignment.Center:
- pt.X = (layoutRectangle.Left + layoutRectangle.Right) / 2f;
- break;
- case StringAlignment.Far:
- pt.X = layoutRectangle.Right - size.Width / 2f;
- break;
- default:
- break;
- }
-
- switch (format.LineAlignment)
- {
- case StringAlignment.Near:
- pt.Y = layoutRectangle.Top + size.Height / 2f;
- break;
- case StringAlignment.Center:
- pt.Y = (layoutRectangle.Top + layoutRectangle.Bottom) / 2f;
- break;
- case StringAlignment.Far:
- pt.Y = layoutRectangle.Bottom - size.Height / 2f;
- break;
- default:
- break;
- }
-
- return pt;
- }
- }
- }
测试代码如下:
[csharp] view plaincopy
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Windows.Forms;
-
- namespace RotateText
- {
- public partial class FormMain : Form
- {
- private Font _font = new Font("Arial", 12);
- private Brush _brush = new SolidBrush(Color.Black);
- private Pen _pen = new Pen(Color.Black, 1f);
- private string _text = "Crow Soft";
-
- public FormMain()
- {
- InitializeComponent();
- }
-
- protected override void OnPaint(PaintEventArgs e)
- {
- base.OnPaint(e);
-
- GraphicsText graphicsText = new GraphicsText();
- graphicsText.Graphics = e.Graphics;
-
-
- StringFormat format = new StringFormat();
- format.Alignment = StringAlignment.Center;
- format.LineAlignment = StringAlignment.Center;
-
- graphicsText.DrawString(_text, _font, _brush, new PointF(100, 80), format, 45f);
- graphicsText.DrawString(_text, _font, _brush, new PointF(200, 80), format, -45f);
- graphicsText.DrawString(_text, _font, _brush, new PointF(300, 80), format, 90f);
- graphicsText.DrawString(_text, _font, _brush, new PointF(400, 80), format, -60f);
-
-
-
- RectangleF rc = RectangleF.FromLTRB(50, 150, 200, 230);
- RectangleF rect = rc;
- format.Alignment = StringAlignment.Near;
-
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, 30);
-
- rect.Location += new SizeF(180, 0);
- format.LineAlignment = StringAlignment.Near;
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, -30);
-
- rect.Location += new SizeF(180, 0);
- format.LineAlignment = StringAlignment.Center;
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, -90);
-
- rect.Location += new SizeF(180, 0);
- format.LineAlignment = StringAlignment.Far;
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, 70);
-
-
- rect = rc;
- rect.Location += new SizeF(0, 100);
- format.Alignment = StringAlignment.Center;
-
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, 40);
-
- rect.Location += new SizeF(180, 0);
- format.LineAlignment = StringAlignment.Near;
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, 30);
-
- rect.Location += new SizeF(180, 0);
- format.LineAlignment = StringAlignment.Center;
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, -70);
-
- rect.Location += new SizeF(180, 0);
- format.LineAlignment = StringAlignment.Far;
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, 60);
-
-
- rect = rc;
- rect.Location += new SizeF(0, 200);
- format.Alignment = StringAlignment.Far;
-
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, -30);
-
- rect.Location += new SizeF(180, 0);
- format.LineAlignment = StringAlignment.Near;
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, -30);
-
- rect.Location += new SizeF(180, 0);
- format.LineAlignment = StringAlignment.Center;
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, 90);
-
- rect.Location += new SizeF(180, 0);
- format.LineAlignment = StringAlignment.Far;
- e.Graphics.DrawRectangle(_pen, rect.Left, rect.Top, rect.Width, rect.Height);
- graphicsText.DrawString(_text, _font, _brush, rect, format, 45);
- }
- }
- }
效果如下图:
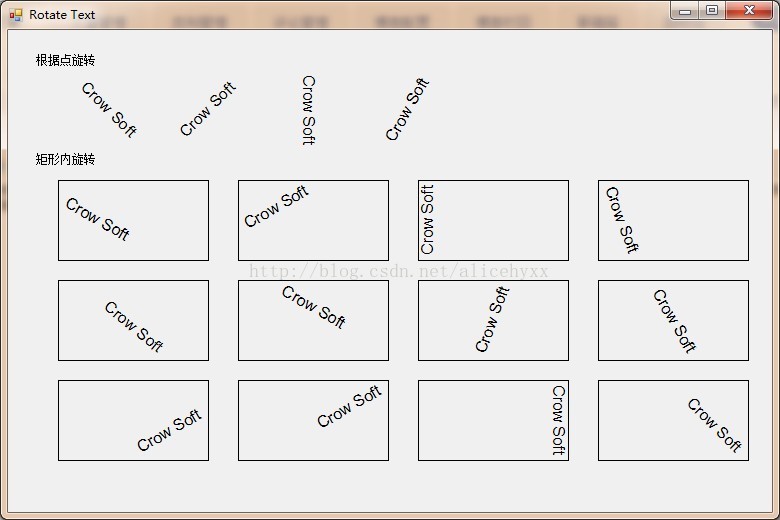
资源地址:http://download.csdn.net/detail/alicehyxx/6626473