模拟主持人发布一个问题,由多个嘉宾来回答这个问题。
分析:从需求中抽出Host (主持人) 类和Guests (嘉宾) 类。
作为问题的发布者,Host不知道问题如何解答。因此它只能发布这个事件,将事件委托给多个嘉宾去处理。因此在Host 类定义事件,在Guests类中定义事件的响应方法。通过多番委托的"+="将响应方法添加到事件列表中,最终 Host 类将触发这个事件。实现过程如下:
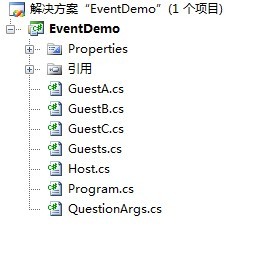
代码其实很少下面贴出来所有代码:
QuestionArgs.cs
view plaincopy to clipboardprint?
class="dp-c">
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- public class QuestionArgs:EventArgs
- {
- public string Message { get; set; }
- }
- }
[csharp] view plaincopyprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- public class QuestionArgs:EventArgs
- {
- public string Message { get; set; }
- }
- }
Program.cs
view plaincopy to clipboardprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- class Program
- {
- static void Main(string[] args)
- {
- Host host = new Host();
- host.Name = "主持人";
- host.args.Message = "C#的事件如何实现的?";
- Guests[] gArray = new Guests[3]
- {
- new GuestA(){Name = "张小三"},
- new GuestB(){Name = "李小四"},
- new GuestC(){Name = "王老五"}
- };
-
- host.QuestionEvent += new QuestionHandler(gArray[0].answer);
- host.QuestionEvent += new QuestionHandler(gArray[1].answer);
- host.QuestionEvent += new QuestionHandler(gArray[2].answer);
-
-
- host.StartAnswer();
- Console.ReadLine();
- }
- }
- }<span style="color:#ff0000;">
- </span>
[csharp] view plaincopyprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- class Program
- {
- static void Main(string[] args)
- {
- Host host = new Host();
- host.Name = "主持人";
- host.args.Message = "C#的事件如何实现的?";
- Guests[] gArray = new Guests[3]
- {
- new GuestA(){Name = "张小三"},
- new GuestB(){Name = "李小四"},
- new GuestC(){Name = "王老五"}
- };
-
- host.QuestionEvent += new QuestionHandler(gArray[0].answer);
- host.QuestionEvent += new QuestionHandler(gArray[1].answer);
- host.QuestionEvent += new QuestionHandler(gArray[2].answer);
-
-
- host.StartAnswer();
- Console.ReadLine();
- }
- }
- }<span style="color:#ff0000;">
- </span>
Host.cs
view plaincopy to clipboardprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- public delegate void QuestionHandler(object sender,QuestionArgs e);
- public class Host
- {
-
- public event QuestionHandler QuestionEvent;
- public QuestionArgs args { set; get; }
- public Host()
- {
-
- args = new QuestionArgs();
- }
- public string Name { get; set; }
- public void StartAnswer()
- {
- Console.WriteLine("开始答题");
- QuestionEvent(this, args);
- }
- }
- }
[csharp] view plaincopyprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- public delegate void QuestionHandler(object sender,QuestionArgs e);
- public class Host
- {
-
- public event QuestionHandler QuestionEvent;
- public QuestionArgs args { set; get; }
- public Host()
- {
-
- args = new QuestionArgs();
- }
- public string Name { get; set; }
- public void StartAnswer()
- {
- Console.WriteLine("开始答题");
- QuestionEvent(this, args);
- }
- }
- }
Guests.cs
view plaincopy to clipboardprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
-
-
-
- public class Guests
- {
-
-
-
- public string Name { get; set; }
-
- public virtual void answer(object sender, QuestionArgs e)
- {
- Console.Write("事件的发出者:" + (sender as Host).Name);
- Console.WriteLine("问题是:" + e.Message);
- }
- }
- }
[csharp] view plaincopyprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
-
-
-
- public class Guests
- {
-
-
-
- public string Name { get; set; }
-
- public virtual void answer(object sender, QuestionArgs e)
- {
- Console.Write("事件的发出者:" + (sender as Host).Name);
- Console.WriteLine("问题是:" + e.Message);
- }
- }
- }
GuestC.cs
view plaincopy to clipboardprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- class GuestC:Guests
- {
- public override void answer(object sender, QuestionArgs e)
- {
- base.answer(sender, e);
- Console.WriteLine("{0}开始答题:我不知道", this.Name);
- }
- }
- }
[csharp] view plaincopyprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- class GuestC:Guests
- {
- public override void answer(object sender, QuestionArgs e)
- {
- base.answer(sender, e);
- Console.WriteLine("{0}开始答题:我不知道", this.Name);
- }
- }
- }
GuestB.cs
view plaincopy to clipboardprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- class GuestB:Guests
- {
- public override void answer(object sender, QuestionArgs e)
- {
- base.answer(sender, e);
- Console.WriteLine("{0}开始答题:我不知道", this.Name);
- }
- }
- }
[csharp] view plaincopyprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- class GuestB:Guests
- {
- public override void answer(object sender, QuestionArgs e)
- {
- base.answer(sender, e);
- Console.WriteLine("{0}开始答题:我不知道", this.Name);
- }
- }
- }
GuestA.cs
view plaincopy to clipboardprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- class GuestA:Guests
- {
- public override void answer(object sender, QuestionArgs e)
- {
- base.answer(sender, e);
- Console.WriteLine("{0}开始答题:我不知道", this.Name);
- }
- }
- }
[csharp] view plaincopyprint?
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
-
- namespace EventDemo
- {
- class GuestA:Guests
- {
- public override void answer(object sender, QuestionArgs e)
- {
- base.answer(sender, e);
- Console.WriteLine("{0}开始答题:我不知道", this.Name);
- }
- }
- }
运行结果:
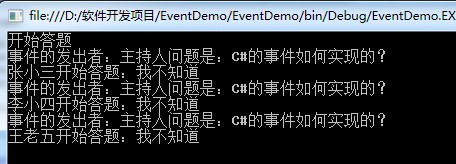