最近要实现android读取sqlite数据库文件,在这里先做一个英汉字典的例子。主要是输入英语到数据库中查询相应的汉语意思,将其答案输出。数据库采用sqlite3.
如图:
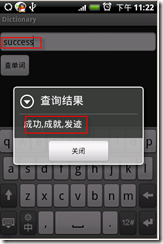
实现过程完全是按照参考文章中所述。其中要说明的是,程序在第一次启动的时候,会把数据库安装到内存卡上面,从而可以读却数据库。
相关的代码:
Java代码
- package com.easymorse;
-
- import java.io.File;
- import java.io.FileOutputStream;
- import java.io.InputStream;
-
- import android.app.Activity;
- import android.app.AlertDialog;
- import android.database.Cursor;
- import android.database.sqlite.SQLiteDatabase;
- import android.os.Bundle;
- import android.text.Editable;
- import android.text.TextWatcher;
- import android.util.Log;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.AutoCompleteTextView;
- import android.widget.Button;
-
- public class Dictionary extends Activity implements OnClickListener, TextWatcher{
- private final String DATABASE_PATH = android.os.Environment
- .getExternalStorageDirectory().getAbsolutePath()
- + "/dictionary";
- private final String DATABASE_FILENAME = "dictionary.db3";
- SQLiteDatabase database;
- Button btnSelectWord;
- AutoCompleteTextView actvWord;
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
-
- database = openDatabase();
-
- btnSelectWord = (Button) findViewById(R.id.btnSelectWord);
- actvWord = (AutoCompleteTextView) findViewById(R.id.actvWord);
- btnSelectWord.setOnClickListener(this);
- actvWord.addTextChangedListener(this);
- }
- public void onClick(View view)
- {
-
- String sql = "select chinese from t_words where english=?";
- Cursor cursor = database.rawQuery(sql, new String[]
- { actvWord.getText().toString() });
- String result = "未找到该单词.";
-
- if (cursor.getCount() > 0)
- {
-
- cursor.moveToFirst();
- result = cursor.getString(cursor.getColumnIndex("chinese"));
- Log.i("tran", "success"+result);
- }
-
- new AlertDialog.Builder(this).setTitle("查询结果").setMessage(result)
- .setPositiveButton("关闭", null).show();
-
- }
-
- private SQLiteDatabase openDatabase() {
- try {
-
- String databaseFilename = DATABASE_PATH + "/" + DATABASE_FILENAME;
- File dir = new File(DATABASE_PATH);
-
- if (!dir.exists())
- dir.mkdir();
-
-
-
- if (!(new File(databaseFilename)).exists()) {
-
- InputStream is = getResources().openRawResource(
- R.raw.dictionary);
- FileOutputStream fos = new FileOutputStream(databaseFilename);
- byte[] buffer = new byte[8192];
- int count = 0;
-
- while ((count = is.read(buffer)) > 0) {
- fos.write(buffer, 0, count);
- }
-
- fos.close();
- is.close();
- }
-
- SQLiteDatabase database = SQLiteDatabase.openOrCreateDatabase(
- databaseFilename, null);
- return database;
- } catch (Exception e) {
- }
- return null;
- }
- @Override
- public void afterTextChanged(Editable s) {
- }
- @Override
- public void beforeTextChanged(CharSequence s, int start, int count,
- int after) {
- }
- @Override
- public void onTextChanged(CharSequence s, int start, int before, int count) {
- }
-
- }